计算机组成 2
Lecture 2 Instructions: Language of the Machine
Outline
- Introduction
- Operations of the computer hardware (计算机硬件的操作)
- Operands of the computer hardware(计算机硬件的操作数)
- Signed and unsigned numbers (有符号和无符号数) 太简单跳过了
- Representing instructions in the computer(计算机中指令的表示)
- Logical operations(逻辑操作)
- Instructions for making decision(决策指令)
- Supporting procedures in computer hardware(计算机对过程的支持) (函数)
- Instruction addressing (指令的寻址)
Operation
design principle 1 Simplicity favors regularity
规范化让操作更简单
简单可以保证低消耗和高性能
32 bits = 1 word
64 bits = double word
Operand
- x0-x31 64bit general registers. design principle 2 Smaller is faster 但是2的倍数永远最优
- 对内存的操作只能通过ld、sd两类指令完成
内存比寄存器慢
翻译器必须尽量使用寄存器 - Little Endian: 小端规则,超过一个word的内容如何排序:小字节放在小的地址(前面),高字节放后面(0x12345678:内存从左至右78 56 34 12)
least significant byte at least address of a word 最低位放在小端
MIPS ARM Alpha SPARC使用大端规则 - Risc-V不需要内存中的word内存对齐(memory alignment)
- RISCV中的立即数
- RISCV R-Format Instruction
function(7b) | RS2(5b) | RS1(5b) | function(3b) | DR(5b) | opcode(7b) |
---|---|---|---|---|---|
0000000 | 00000 | 00000 | 000 | 00000 | 0000000 |
design principle 3 Good design demands good compromises
不同的格式会增大decoding的难度,但是要允许这类情况
尽量保持指令格式相同
- RISCV S-Format Instruction
imm[11:5] | RS2 | RS1 | function | imm[4:0] | opcode(7b) |
---|---|---|---|---|---|
7b | 5b | 5b | 3b | 5b | 0000000 |
design principle 3 Good design demands good compromises
现阶段计算机两大principle
指令被当作数字
程序被当作数字存在内存中——冯诺依曼架构指令集结构的几种类型
R-type 三个R
I-type 两个R一个立即数
S-type
SB-type
UJ-type
U-type
Logical Operations
- Instructions for bitwise manipulation
Operation | RISCV |
---|---|
Shift left logical | slli |
Shift right | srli |
Bit-by-bit AND | and, andi |
Bit-by-bit OR | or, ori |
Bit-by-bit XOR/NOT | xor, xori |
- RISC-V assembly language of logical and shift
- logical:
and/or/xor/andi/ori/xori dr, sr1, sr2 - shift:
sll/srl/sra/slli dr, sr1, sr2
Instructions for making decisions
Branch instructions
- beq r1, r2, Location 相等就跳转到 Location
- bne r1, r2, Location 不等就跳转到 Location
An Example of
if else then
clausebne r1,r2. ELSE
things to do if
beq x0,x0, EXIT
ELSE: else do
EXIT: exit
Supports
LOOPS
LOOP: ......
add x22,x22,x23(x22++)
bne x22,x21,LOOP
Suport
while
- 和loop类似。区别:开头一个exit的判断和跳转,loop末尾一个铁定的jump
set on less than : slt
- slt x5, x19, x20 #
set x5=1 if x19 < x20
- slt x5, x19, x20 #
other conditional operation
- blt rs1,rs2,L1 #
if(rs1<rs2)branch to instruction L1
- bge rs1,rs2,L1 #
if(rs1>=rs2)branch to instruction L1
- bltu bgeu: Unsigned Comparison
- blt rs1,rs2,L1 #
Hold out Case/Switch:
很诡异,只有case0123这样才会支持
jalr r1,imm(r2) 跳到r2加上偏移量,然后将下一句话的地址存到r1Important conception–Basic Blocks
- 除了结尾之处,没有内置分支
- 除了开始之处,没有分支标签
Supporting Procedures in Computer Hardware 如何支持函数调用?
传入参数到一个函数可以访问的地方 (argument)
把控制权交给进程(jump到那个位置)
得到寄存器的储存资源(原来的值暂存)
做想做的事情
把算好的结果放到主函数能接触到的地方
返回到原先的位置Procedure Call Instructions
Caller使用 jal 指令
jal指令: jump and link jal x1, ProcedureAddress
把下一个指令的位置(要返回的位置)放到x1
然后条到 ProcedureAddress的位置Callee使用 jalr 指令
jalr指令:jump and link register jalr x0,0(x1)
把下一条指令的位置放到x0(实际啥也没干)
然后跳到x1+0的位置Using More Registers
More Registers for procedure calling:
- a0 ~ a7(x10-x17): eight argument registers to pass parameters & return values
- ra/x1:one return address register to return to origin point
Stack:
- ideal data structure for spilling registers
- Push, pop , Stack pointer ( sp )
Pointer of stack
$fpsaved argument registers saved return address saved saved resisters Local variables
$sp
内存中的映射:(地址从上往下由高到低)
static data是全局变量
Text是程序代码
Dynamic data 是malloc开的heap
stack是自动存储的栈Byte / Halfword / Word Operation
- 1 byte = 8 bits
- 1 Halfword = 2 Byte
- 1 Word = 4 Byte
- 1 Doubleword = 8 Byte
- the offset of the instruction is used by Byte
- lb,lh,lw,ld 这几个要sign extend to 64 bits
- lbu lhu lwu ldu 这几个要0 extend to 64 bits
# 我宣布我已经领略到汇编语言的真谛了 |
Addressing Method
- RISCV fot 32 bit Immediate and Address (Wide bit addressing)
- use instruction lui:
lui s3,976
(高20位放到s3里面)U format addi s3,s3, imm12
低12位对s3加
- use instruction lui:
- Branch Addressing
- SB\Uj format 在后面都补一个0,寻址能力*2
- (只有condition branch(SB)类型和unconditional jump(UJ)类型在后面加0,ldsd都不加)
- Jump Addressing
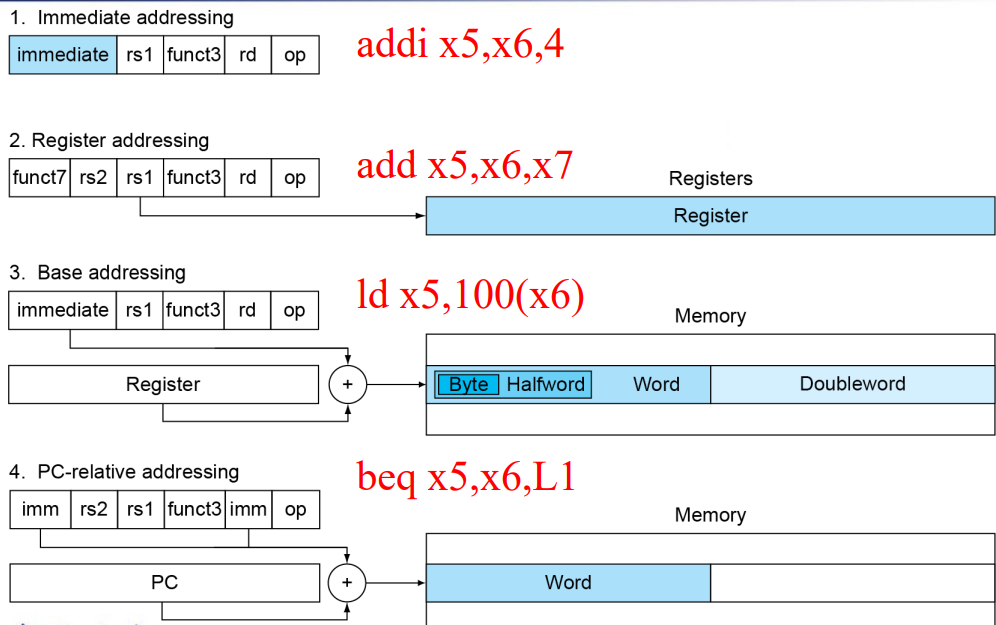
Synchronization in Risc-V
- Use Lock and atomic operation:
- Load reserved: lr.d rd,(rs1)
- Store conditional: sc.d rd,(rs1),rs2